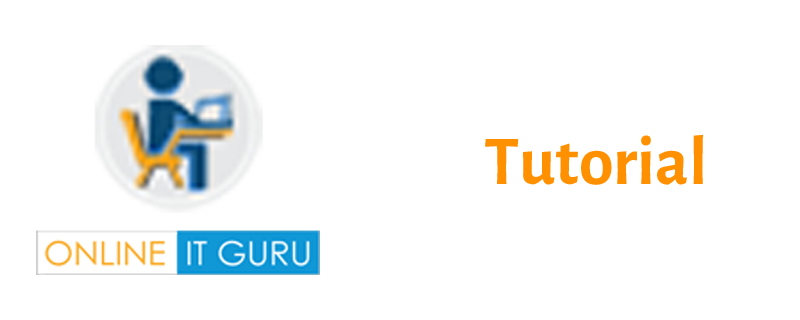
Spring AOP:
Aspect Oriented Programming (AOP) compliments OOPs as in it additionally gives particularity. Be that as it may, the key unit of seclusion is angle than class. Spring AOP breaks the program rationale into unmistakable parts (called concerns). It is utilized to build particularity by cross-cutting concerns.
A cross-cutting concern is a worry that can influence the entire application and ought to be brought together in one area in code as could be allowed, for example, exchange administration, confirmation, logging, security.
There are 5 techniques that begins from m, 2 strategies that begins from n and 3 strategies that begins from p.To get in-depth knowledge on Java, you can enroll for live Java online course by OnlineITGuru with 24/7 support and lifetime accessUnderstanding Scenario I need to keep up log and send warning in the wake of calling strategies that begins m.Issue without AOP We can call techniques (that keeps up log and sends notice) from the strategies beginning with m. In such situation, we have to compose the code in all the 5 strategies.AOP Concepts and Terminology
- Join point
- Advice
- Pointcut
- Introduction
- Target Object
- Aspect
- Interceptor
- AOP Proxy
- Weaving
- AspectJ
- Spring AOP
- JBoss AOP
There are 4 sorts of advices maintained in spring1.2 old style aop utilization.
Before Advice
After Advice
Around Advice
- Throws Advice
hierarchy of advice interfaces:
MethodBeforeAdvice interface extends the BeforeAdvice interface.
AOP AspectJ Annotation:
The Spring Framework prescribes you to utilize Spring AspectJ AOP execution over the Spring 1.2 old style dtd based AOP usage since it gives you more control.
- By annotation: We are going to learn it here.
- By xml configuration (schema based)
Understanding Pointcut:
The @Pointcut comment is utilized to characterize the pointcut. We can allude the pointcut articulation by name too. How about we see the straightforward case of pointcut articulation.
@Pointcut("execution(* Operation.*(..))")
private void doSomething() {}Understanding Pointcut Expressions
@Pointcut("execution(public * *(..))")@Pointcut("execution(public Operation.*(..))")@Pointcut("execution(* Operation.*(..))")@Pointcut("execution(public Employee.set*(..))")@Pointcut("execution(int Operation.*(..))")AOP AspectJ Xml Configuration
Spring empowers you to characterize the perspectives, advices and pointcut in xml record. In the past page, we have seen the AOP cases utilizing explanations. Presently we will see same cases by the xml design record.- aop:before
- aop:after
- aop:after-returning
- aop:around
- aop:after-throwing
aop:before Example
File: Operation.java
package com.javatspot;public class Operation{public void msg(){System.out.println("msg method invoked");}public int m(){System.out.println("m method invoked");return 2;}public int k(){System.out.println("k method invoked");return 3;}} File: TrackOperation.javapackage com.javaspot;import org.aspectj.lang.JoinPoint;public class TrackOperation{public void myadvice(JoinPoint jp)//it is advice{System.out.println("additional concern");//System.out.println("Method Signature: " + jp.getSignature());}} File: applicationContext.xmlOutput
calling msg...additional concernmsg() method invokedcalling m...additional concernm() method invokedcalling k...additional concernk() method invoked2) aop:after example File: applicationContext.xmlOutput
calling msg...msg() method invokedadditional concerncalling m...m() method invokedadditional concerncalling k...k() method invokedadditional concern3) aop:after-returning example
File: Operation.javapackage com.javaspot;public class Operation{public int m(){System.out.println("m() method invoked");return 2;}public int k(){System.out.println("k() method invoked");return 3;}}Create the aspect class that contains after returning advice.File: TrackOperation.javapackage com.javaspot;import org.aspectj.lang.JoinPoint;public class TrackOperation{public void myadvice(JoinPoint jp,Object result)//it is advice (after advice){System.out.println("additional concern");System.out.println("Method Signature: " + jp.getSignature());System.out.println("Result in advice: "+result);System.out.println("end of after returning advice...");}} File: applicationContext.xmlOutput
calling m...m() method invokedadditional concernMethod Signature: int com.javaspot.Operation.m()Result in advice: 2end of after returning advice...2calling k...k() method invokedadditional concernMethod Signature: int com.javaspot.Operation.k()Result in advice: 3end of after returning advice...34) aop:around example
The AspectJ around advice is applied before and after calling the actual business logic methods.Create a class that contains actual business logic.File: Operation.javapackage com.javaspot;public class Operation{public void msg(){System.out.println("msg() is invoked");}public void display(){System.out.println("display() is invoked");}}.File: TrackOperation.javapackage com.javaspot;import org.aspectj.lang.ProceedingJoinPoint;public class TrackOperation{public Object myadvice(ProceedingJoinPoint pjp) throws Throwable{System.out.println("Additional Concern Before calling actual method");Object obj=pjp.proceed();System.out.println("Additional Concern After calling actual method");return obj;}} File: applicationContext.xmlOutput
Additional Concern Before calling actual methodmsg() is invokedAdditional Concern After calling actual methodAdditional Concern Before calling actual methoddisplay() is invokedAdditional Concern After calling actual method 5) aop:after-throwing example File: Operation.javapackage com.javaspot;public class Operation{public void validate(int age)throws Exception{if(age<18){throw new ArithmeticException("Not valid age");}else{System.out.println("Thanks for vote");}}} File: TrackOperation.javapackage com.javaspot;import org.aspectj.lang.JoinPoint;public class TrackOperation{public void myadvice(JoinPoint jp,Throwable error)//it is advice{System.out.println("additional concern");System.out.println("Method Signature: " + jp.getSignature());System.out.println("Exception is: "+error);System.out.println("end of after throwing advice...");}}File: applicationContext.xmlOutput
calling validate...Thanks for votecalling validate again...additional concernMethod Signature: void com.javaspot.Operation.validate(int)Exception is: java.lang.ArithmeticException: Not valid ageend of after throwing advice...java.lang.ArithmeticException: Not valid ageJdbcTemplate:
Spring JdbcTemplate is an effective instrument to associate with the database and execute SQL inquiries. It inside utilizations JDBC programming interface, however dispenses with a great deal of issues of JDBC API.Issues of JDBC APIThe issues of JDBC API are as per the following:We have to compose a ton of code previously, then after the fact executing the question, for example, making association, proclamation, shutting resultset, association and so forth.We have to perform special case taking care of code on the database logic.We have to deal with exchange. Reiteration of every one of these codes starting with one then onto the next database rationale is a tedious assignment.Preferred standpoint of Spring JdbcTemplate Spring JdbcTemplate wipes out all the previously mentioned issues of JDBC API. It gives you strategies to compose the inquiries straightforwardly, so it spares a great deal of work and time.Spring Jdbc Approaches
- JdbcTemplate
- NamedParameterJdbcTemplate
- SimpleJdbcTemplate
- SimpleJdbcInsert and SimpleJdbcCall
JdbcTemplate class
It is the focal class in the Spring JDBC bolster classes. It deals with creation and arrival of assets, for example, making and shutting of association question and so forth. So it won't prompt any issue on the off chance that you neglect to close the association.It handles the special case and gives the useful exemption messages by the assistance of excepion classes characterized in the org.springframework.dao bundle. We can play out all the database operations by the assistance of JdbcTemplate class, for example, addition, updation, cancellation and recovery of the information from the database.No. | Method |
1) | public int update(String query) |
2) | public int update(String query,Object... args) |
3) | public void execute(String query) |
4) | public Texecute(Stringsql, PreparedStatementCallback action) |
5) | public T query(String sql, ResultSetExtractorrse) |
6) | public List query(String sql, RowMapper rse) |
PreparedStatement in Spring JdbcTemplate:
Syntax :
public T execute(String sql,PreparedStatementCallback);
PreparedStatementCallback interface
The input parameters and output results, you don't need to care about single and double quotes.Method of PreparedStatementCallback interface
It has only one method doInPreparedStatement- publicT doInPreparedStatement(PreparedStatement ps)throws SQLException, DataAccessException
ResultSetExtractor
We can without much of a stretch get the records from the database utilizing inquiry() strategy for JdbcTemplate class where we have to pass the example of ResultSetExtractor.Syntax- publicT query(String sql,ResultSetExtractor
rse)
- publicT extractData(ResultSet rs)throws SQLException,DataAccessException
RowMapper
Syntax
- publicT query(String sql,RowMapper
rm)
- publicT mapRow(ResultSet rs, int rowNumber)throws SQLException
Spring NamedParameterJdbcTemplate
Spring gives another approach to embed information by named parameter. In such way, we utilize names rather than ?(question mark). So it is smarter to recall the information for the segment.Basic case of named parameter inquiryinsert into employee values (:id,:name,:salary) Strategy for NamedParameterJdbcTemplate classIn this example,we will call just the execute strategy for NamedParameterJdbcTemplate class. Grammar of the technique is as take after.Spring SimpleJdbcTemplate
spring 3 JDBC underpins the java 5 highlight var-args (variable contention) and autoboxing by the assistance of SimpleJdbcTemplate class.SimpleJdbcTemplate class wraps the JdbcTemplate class and gives the refresh technique where we can pass subjective number of contentions.Syntax of update method of SimpleJdbcTemplate class
- intupdate(String sql,Object... parameters)