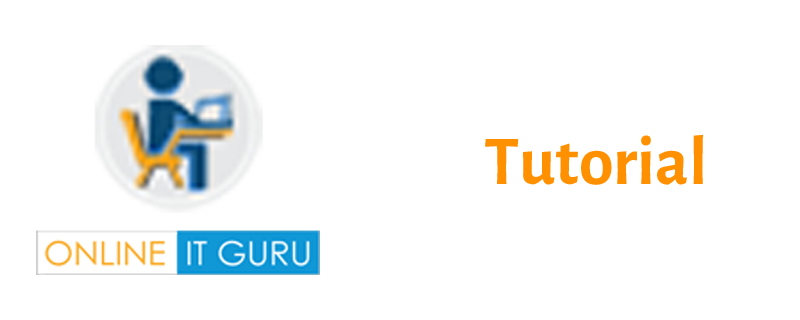
Since as discussed in the python definition, Python is an Object-oriented programming language. So, now its time to discuss Classes and objects.
Class:
Everything in python is considered as a class. It is a user-defined prototype for the object. It defined the set of attributes defines the attributes of the class. The attributes are the data members. We can access these methods using dot (.) notation
We can define the class with Class followed by the class name. The class name should be followed by colon (:)
Class Class_name(): StatementsPrint(Class_name)Ex:class A: x = 5 print (x)print(A)Output :5
Object:
It is a unique instance of the data structure. Usually class defines the objects. An object comprises both data methods and members.
Method :
It is a special kind of function, that is defined in the class definition.
Data member :
These can be class variables (or) the instance variables. They hold the data, that is associated with the class and objects.
Instance :
An instance is an individual object of a certain class. For example, an object belongs to the class circle, would be an instance on the class circle.
Class variables :
It is a variable that can be shared via class instances. These variables are declared within the class but outside the methods. But these are not frequently used as instance variables.
Instance Variables :
Inside method, we can define variables. These variables belong to the current class instance.
Python With oop:
Before we were going to discuss python with oop, let us first have a look on
Why object-oriented programming (oop)?
Programming languages were basically classified into two types. Procedural (or) structural programming language and object-oriented programming. In object-oriented programming, a program has several mini-programs. Here each object represents a different part of the application. And each part of the application has its own logic. This helps to communicate among themselves.
Now, lets have a look over python object oriented features.
Abstraction:
To simplify the code complexity abstraction is used . But we cannot instantiate the abstract class. It means for these classes, we cannot create the objects (or) classes. We can abstraction to inherit certain functionalities. Moreover, here we can inherit the functionalities, but at the same time, we cannot instance of the particular class.
Since this property is common in OOP like JAVA, here I would like to leave the code for you as an assignment. If you need any help feel free to contact python online education
Inheritance :
This is basically the property of the class. Through this concept, we can transfer one class properties to the other. This is useful at the time of subclass creation. These sub classes can easily get the properties from the parent classes. Moreover , as per the client requirement, without affecting the parent functionality, here we can override (or) add the new functionalities.
So, to use this inheritance, we must have two classes.
a)Parent class(Base(or) superclass)
b)Child class(Subclass(or) derived class)
Usually, the child class inherits the parent class properties. This Inheritance is further divided into types. Those are single, multilevel, hierarchical and multiple inheritances. Let us discuss one by one in detailed.
Single Inheritance :
A class that is inherited from another single parent class itself is called single inheritance
Multiple inheritances:
It means you are inheriting the properties of multiple classes into one. In this, two classes A and B inherits the properties from Class C.
Multilevel inheritance :
In this level of inheritance, various properties were acquired at various levels.
Hierarchical Inheritance:
Functional overloading :
It means assigning more than a single behavior to a particular behavior. The operation here performed varied by the argument types and the objects involved.
Operator overloading :
It means assigning more than one function to the particular operator.
Attributes in Python :
It defines the property of the object. element (or) file. Python supports two types of attributes.
Built-in class attributes :
These are the built-in attributes present inside the python classes. For example, _dict_, _doc_, _name_ etc. Let me consider the attributes with an example.
Let us assume, we have the below table
Now, I would like to display the employee_1 details. All the details that belong to employee_ 1 are known as Attributes of the employee.
We can achieve this as follows
This would display the following output.
‘First_name’:’Balajee’,’ Last_name’: ‘Nanduri’, ‘salary’:25000
User-defined attributes :
We can create these attributes inside the class definition. Additionally, Python allows you to dynamically create the new classes for the existing class instances.Like C and JAVA, this python attributes also have the variable scope. Let us have a look over those in detailed.
Scope of the attributes :
Public:
We can use these attributes class definition inside (or) outside
Protected :
We can use these attributes inside the subclass definition, but not outside the class definition.
Private :
This kind of attribute is inaccessible and invisible. Except inside the class definition, it is neither possible to read nor possible to write those definitions.
Polymorphism:
It is the ability to present the same interface in different underlying forms. Technically speaking, if the child class inheriting the parents it does not necessarily mean to inherit about the parent class. In other words, unlike parent class, it may do some things in a different manner. Basically, polymorphism is applied using inheritance. Moreover, IT training says python is implicitly polymorphic. It means python has the ability to override the standard operators.
Let the get the more clarity on this with the example below:
class cow(object): def color(self): print("white")class buffalo(object): def color(self): print("black")def animalcolor(animalType): animalType.color()cowObj = cow()bufObj = buffalo()animalcolor(cowObj)animalcolor(bufObj) Output :whiteblack