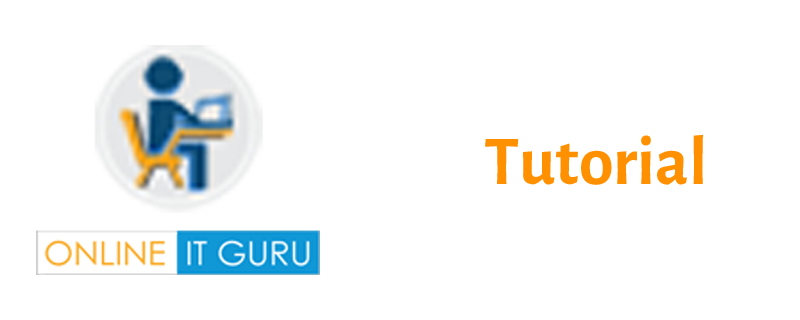
And the next topic, that I would like to discuss is Errors and exceptions in python?
Errors and exceptions in python:
Even this concept is familiar in other programming languages like JAVA, it’s the best thing to recall those terms once.
What is an error?
An error is a condition that occurs when the programme does not follow the proper structure.
Basically, errors are classified into two types :
Syntax errors and
Logical errors
Syntax errors:
These errors occur if the code violates the compiler (or) interpreter rules
For example:
test = [1,2,3]
for i in the test:
print('This is an error')#here we have an indentation error
Output:
IndentationError: expected an indented block.
So the next thing, that we need to discuss is about the exceptions
What is an exception?
These are nothing but the logical errors that were found during run time. In other words, if the program is compiled (or) interpreted the operation (Compilation/interpretation) goes well. But soon after, at run time we may found expectations. These are nothing but exceptions.
Division with zero is an example of an exception.
Get the best real time examples of exceptions at online certificate courses
Python interpreter supports some built-in exception. let us have look over those.
Exception Class | Event |
I/O errors | It arises when the input/ output operation fails |
Arithmetic error | It arises when the numeric calculation fails |
Floating point error | It arises when the floating point calculation fails |
Zero division error | It arises when the division (or) modulo by zero occurs |
Assertion error | It arises when the assert statement fails |
Overflow error | It arises when the arithmetic operation is too large |
Import error | It arises when the imported module is not found |
Keyboard interrupt error | It arises when the user interrupts the process execution. It is usually was done by pressing Ctrl+c |
Stop iteration error | It raises when the next method of the iterator does not point to any object |
Indentation error | It arises when there is an incorrect indentation |
Name error | It arises when the identifier is not found in the local (or) global namespace |
Key error | It arises when the specified key is not found in the dictionary |
Value error | It arises when the function gets arguments of correct type but improper value. |
Attribute error | It arises when the attribute reference (or) assignment fails |
Syntax error | It arises by the parser when the syntax error is encountered |
Index error | It arises when the index is out of range |
Runtime error | It arises when the generated error does not fall into any category |
Type error | It arises when the function is applied to the object of incorrect type |
Unbound local error | It arises when we are trying to access the local variable (or) a method in a function. But here default value is not assigned. |
EOF error | It arises when there is no input either from raw _input() (or) input function |
So likewise python supports many built-in functions. Visit it certifications to get examples on these.
In real time, we cannot expect the code in a few lines. The program should not stop executing for an unexpected (Unknowing error), the program should not stop executing. In such a case, we need an alternative for the execution of the programs. Try – raise exception provides a solution to this kind of problems.
So let us have a look what does these mean.
Try:
In python using try. we can catch exception. But, If any code within the try causes an exception, exception of the code will stop and jump to the except statement. But When an exception occurs in the try block, python looks for the matching except block to handle it.
Syntax:
try:
statements
except:
statements
statements
Example:
try:
x > 100
except:
print("Something went wrong")
print("Even if it raised an error, the program keeps running")
Output :
Something went wrong
Even if it raised an error, the program keeps running.
The one more kind of exception that we need to handle is the try-except block:
Here in the try block, we can place the suspicious code where there is a probability of error. After the try block, we can place the except statement. The except statement is followed by the block of code. This handles the code as efficiently as possible. The syntax is shown below:
Syn:
try:
statements
except exceptions 1
--------------------
---------------------
Except exceptions 2
…………
………….
else:
if there is no exception, this block is executed.
Example :
try: ab = open("example", "r") ab.write("This is my test file for exception handling!!")except IOError: print ("Error: can\'t find file or read data")else: print ("Written content in the file successfully")Output :Error: can't find file or read data
Features of try-except blocks :
A single try block has multiple except statements. This is useful when the try block contains exceptions and throws different exceptions.
Here you can provide a generic except clause which handles any exception
After the except clause, we can include an else clause. If the code in the try block does not raise an exception the code in the else block executes.
The else block is a good place for the code which does not require the try- block protection.
Can we use except class without exceptions?
Yes, we can use the except class where there is no exception defined. Usually, the try-catch statement catches all the exception that occurs. Because in the good programming practice, try- except is not considered as a best. Besides, the try-except statement catches all the exceptions. But it does not make the programmer identify the root cause of the problem.
Note:
We can use the same except statement to handles multiple exceptions.
Syn:try: Operations ......................except(Exception1[, Exception2[,...ExceptionN]]]): This block executes If there is an exception from the given exception list, ......................else:
This block executes only If there is no exception
And the last one that we need to discuss here is try – finally, clause:
As said many times, we cannot except the code to be alike. There might be situations where the programmer need to execute the group of statements compulsorily( irrespective) In such a condition, we would use try- finally.
Python interpreter allows you to use finally along with try. Irrespective of the condition satisfies, it is a piece of code that must execute mandatory.
try:
error possibility area
......................
This may be skipped due to any exception
finally:
This block of statements will definitely executes.
Example:
With this syntax, let us have a look over the code
try: fh = open("testfile", "r") try: fh.write("This is the example of exception handling with try and finally") finally: print ("I will definately exceutes") fh.close()except IOError: print ("Error: can\'t find file or read data")Output:I will definately exceutesError: can't find file or read data I will definately exceutes
Does an exception allow arguments?
Yes, an exception can have an argument. This gives additional information about the problem. The contents of the arguments varies by an exception. And we can capture an exception argument by supplying a variable in the except clause.
Syntax:
try:
some operations will be done here
......................
except ExceptionType, Argument:
here we can print the argument value.
So like above are you expecting an example? if yes, I would like to say write the code, compile and run it as an assignment. And if you are unable to solve then get the answer from learn python 3.
So till now, we have seen what is an exception, what are the type of exceptions and so on? But have you ever think about
How to raise an exception?
Using the raise statement, we can raise an exception in several ways. The syntax of the raise statement is shown below:
Syn:
Raise [Exception [,args [,traceback]]]
Explanation:
The exception is a type of exception(for example, NameError)
The argument is the argument type value.This is an optional value.
The traceback object is used for an exception. This is also optional.
Example:
try: raise NameError('Hello')except NameError: print('This is an example of raising an error') raiseOutput:Traceback (most recent call last):This is an example of raising an error File "C:/Users/BALU/PycharmProjects/firstproject/first.py", line 2, inraise NameError('Hello')NameError: Hello
Process finished with exit code 1
And the last topics that we need to discuss here is the user defined exceptions
Till now, we have seen the system defined exceptions. But rather than this, a user can also define exceptions. Now let us have a look over
User-defined exceptions:
Python allows the use the create and define exception of our own. Let us have look how to create the user define exceptions.
Example:
# define Python user-defined exceptionsclass Error(Exception): """Base class for other exceptions""" passclass minerror(Error): """Raised when the input value is too small""" passclass maxerror(Error): """Raised when the input value is too large""" pass# our main program# user guesses a number until he/she gets it right# you need to guess this numbernumber = 20while True: try: i_num = int(input("Enter a number: ")) if i_num < number: raise minerror elif i_num > number: raise maxerror break except minerror: print("This value is less than the initialized number, try again!") print() except maxerror: print("This value is greater than the initialized number, try again!") print()print("Congratulations! You guessed it correctly.")Output:Enter a number: 5This value is less than the initialized number, try again!Enter a number: 100This value is greater than the initialized number, try again!