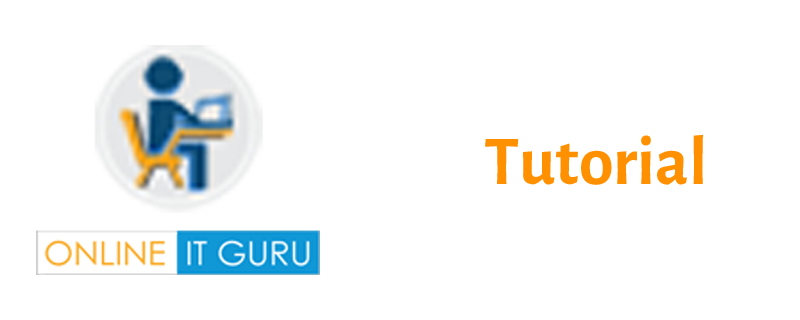
Since python supports code re usability, many people today love to learn python programming. One way to achieve code re usability is FUNCTION(). But with function, we can achieve code re usability for a certain line of code. But there may be a case, where we need to include code in terms of hundreds of lines. In such cases, this function does not work well(Increases the length of the code). So in this case, we will place this code in a separate file and we can use this file at the time of requirement. And this can be achieved through the concept of python modules.
What is a module?
A module is a python file containing Python definitions and statements. The file name should have an extension of .py. In python modules, we can group similar data. This makes the programmer to easily understand the code. And within the module, the module name is available the global variable __name__. This module contains the executable statements as well as the function definitions. Modules can import the other modules too. Usually, modules are imported as follows:
From – This is used to indicate the file names that should be imported from.
Import – this is used to import the entire module
Reload – It permits to reload the python modules. When the module is imported into the script, the code in the top level portion executed only once. And if you wish re-execute this top-level code, you can use the reload ().Moreover, it reloads and imports the function again
reload(module_name)
Note :
But while importing these files, the developers do not require to support .py extension.
Python cannot import the statements started with an underscore(_).
In learn python, now its time to know ,
How to create a module?
AS said above, this python module is a separate python file. So, for example, create a python file, with the name primary. This contains the following code.
import primaryprimary.modules('hero')Now create another python and import this primary.pydef modules(name): print("Hi, " + name)let us save this file as a second.py. So now, we will be getting the output as Output:Hi, hero
Note:
You can use any python import statement in some other python file. This has the following syntax:
Ex: import module1[,module2[,….module N]
Import * statement :
Python interpreter allows you to import all the names from the module into the current namespace. Usually * is used to import all the items into the namespace.
So now you people will be thinking of
How does the python search the file in the directory?
When the python modules are imported, the interpreter is imported in the following sequence:
- Current directory
- If the module is not found, then it searches each directory in the shell variable PYTHONPATH
- And if fails then the python interpreter checks for the default path. In Unix based system, this path is usually found at /usr/local/lib/python.
The module search path is stored in the system module as the sys.path variable. The syspth contains the current directory, the python path, and the installation path.
What is a python path variable?
The python path is an environment variable consists of a list of directories. And the pythonpath syntax is the same as shell variable path
Usually, in the Windows system, the python path system is located at:
set PYTHONPATH = c:\python20\lib;And in the Unix system, the python path is located at :set PYTHONPATH = /usr/local/lib/python
In the best way to learn python, the next topic, that needs to discuss is namespaces and scoping
Namespaces:
Variables are the names that map to the objects. A namespace is a variable name dictionary and their corresponding objects.
A python statement can access the variables in the local namespace as well as the global namespace. And if the local variable and the global variable have the same name, the local variable shadows the global variables.
Each function has its own local namespace. As ordinary functions, the class methods follow the same scoping rules.
Usually, python makes an educated guess on whether variables are local (or) global. It assumes any value assigned is local.
So, within a function, initially, we must use the global statement to define a global variable.
If the statement contains the global varname, then the python interpreter confirms that is a global variable. And it won't search the variable name in the local namespace.
So as said above, this python module consists of several variables, modules, and functions. And the interested contains several built-in packages. And we cannot which module contains which files. So, have you ever thought
How to get the directories and file names in the package?
This can get with the dir(). This function returns all the files and directory names in the package.
import mathcontent = dir(math)print (content)
Output:
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'pi', 'pow', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc']
Besides the dir() , the python interpreter contains several buit- in modules . So let have a look over those built- in modules.
Function | Description |
ceil(n) | Returns the given number next integer |
floor(n) | Returns the previous number of the given number |
sqrt(n) | Returns the square root of the number |
exp(n) | Returns the natural logarithm ‘e’ raised to the power ‘n’ |
log(n.baseto) | Return s the natural logarithm of the number |
power(base to, exp) | Returns base to raised to the exp power |
tan(n) | Returns the tangent of the given radian |
sin(n) | Returns the sine of the given number |
cos(n) | Returns the cosine of the given number |