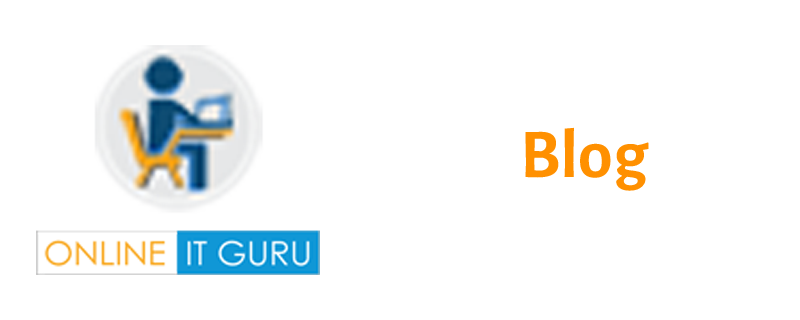
Python programming language includes many functions, methods, libraries that are much useful for coders, users of python, etc. In this way, the Python return statement is a very useful function within Python programming. This is generally useful to check the return values from a function. In other words, the Python return statement is useful to close the execution of the call function. And it returns the outcome or value back to the caller. The statements after the return statements cannot perform. In case, the return statement doesn’t contain any expression, then the special value returned as “None”.
The syntax could be like below for this.
def fun(): |
Python return statement example
We can execute certain operations within a function and return the outcome to the caller using the return statement.
The example (syntax) for the Python return statement is as follows.
def add(x, y): |
Let us discuss in detail the following things in this blog on Python return Statement;
- Using Python return Statement in functions
- Returning single or multiple values from functions
- Best practices of using Python return Statement
Using Python return Statement in functions
Most coding languages allow users to allot a name to a code block that executes a strong computation. Programmers often called these named code blocks as routines, procedures, or Functions depending upon the language. These functions are reusable quickly because users can use their names to call them from multiple places within the code.
In other coding languages, there is a clear difference between a routine, procedure, and function.
Get practical knowledge of Python return statement with hands-on experience with expert advice via the Python Online Course at Onlineitguru.
Sometimes that difference is so concrete that users need to use a particular keyword to explain a procedure or/and another keyword to describe a function. For example, the VB coding language uses Sub and Function to separate between the two.
Typically, a “procedure” is a named code block that executes collective actions without computing a final value or outcome. In this way, a function is a named code block that executes a few actions with the purpose of calculating a final value or outcome. Then this is sent back to the caller code. Thus, both functions & procedures can act upon collective input values, commonly known as “arguments”.
Python return Statement: Function Call
Generally, a function takes arguments, performs some activities, and returns a value as an outcome. This value returned by a function to the caller is usually known as the function’s return value. Moreover, all Python functions within python return Statement (s) include a return value, either explicit or implicit.
To explain a “function” within Python, users can use the below syntax for example:
def function_name(arg1, arg2,..., argN): |
When a user is coding a Python function he needs to explain a header with the def keyword, the name of the function, and a list of arguments in parentheses (). Then users need to describe the function’s code block and it will start a level of indentation towards the right.
To make a function useful, one needs to call it. Besides, a function call includes the function’s name followed by the function’s arguments in (). The first line of the above syntax defines the example of a “function’s call”.
Explicit return Statement
An explicit return statement within Python return Statement right away removes a function performance and sends the return value back to the caller code. To add an explicit return statement to a Python function, the user has to use return followed by an optional return value:
>>> def return_42(): |
For example,
When a user describes return_42(), he adds an “explicit return statement” at the end of the function’s code block. The value 42 within the statement is the explicit return value of return_42(). It refers that any time a user calls return_42(), the function sends back 42 to the caller.
In case, a user defines a function using an “explicit return statement” that includes an explicit return value. Then he can use that return value in any other expression, such as;
>>> num = return_42() |
Since the code “return_42()” returns a numeric value, the user can use this value as a math expression. Moreover, it is useful as any other type of expression wherein the value has a logical meaning. In this way, a caller code can take the benefit of a function’s return value.
Implicit return Statement
A Python function expression will always include a return value. Moreover, there is no concept of any procedure or practice within Python. So, if the user doesn’t clearly use a return value within a Python return statement. Otherwise, he totally excludes the return statement then Python will implicitly return a default value for the user. Besides, this default return value will always be expressed as “None”.
For instance:
A user writes a function that adds 1 to a number N, but he forgets to provide a return statement. In this case, the user will get an implicit return statement that uses “None” return value.
The syntax for the same is as follow
In case, a user doesn’t furnish an “explicit return statement” with a clear return value. Then Python contributes an implicit return statement using “None” as an output.
def add_one(x): |
Python return Statement multiple values
Here, users can use a Python return statement to return multiple values through a function. To perform this, users just need to provide fewer return values separated by commas.
For E.g. users need to write a function that includes a numeric value and returns a summary of statistical estimates. The following syntax helps us to understand it easily.
import statistics as st |
In the describe() function, users take the benefit of Python’s ability to return multiple values within a single return statement. It does so by returning the mean, median, and mode values of the sample in parallel.
Python return Statement: Return type “tuple”
>>> sample = [10, 2, 4, 7, 9, 3, 7, 8, 6, 8] |
Here, a user unpacks the three return values of describe() expression into the 3 variables mean, median, and mode. Here, it converts the return values into a useful manner. Note that in the last example, the user has stored all the values within a single variable, “desc”. This variable turns out to be a Python tuple.
As a note: In order to get or to return multiple values, users just need to write them in a comma-separated list.
||{"title":"Master in Python", "subTitle":"Python Certification Training by ITGURU's", "btnTitle":"View Details","url":"https://onlineitguru.com/python-online-course","boxType":"demo","videoId":"Qtdzdhw6JOk"}||
Return multiple values with “List Display”
A “list display” produces the latest list object, where the contents are stated by a list of expressions. When a comma-separated list of expressions is provided, its elements are assessed from left to right and set into the list object in order. The type of return is a list that is expressed in the following way.
def calc(p,q): |
‘Returning’ vs. ‘Printing’ statements within a function
In this statement, the Python return statement will end the function call and return the value to the caller.
The “print” statement is useful only to print the value. Users can’t allot that result to another variable or pass the output to another function expressly.
For example: Allotting a return value to a variable and also passing it as an argument to another function. This describes the below syntax.
def add(p,q): |
Print statement function
The expression is stated below in the form of syntax.
def add(p,q): |
Python return Statement: Best Practices
Thus, we learned the basics of how the Python return statement works and its examples. Now you got the idea of how to write functions that return one or different values to the caller. In addition, you also got knowledge that if you don’t add an “explicit return statement” with a clear return value to the function stated, then Python will add it for you. That value will be considered “None”.
This section of the blog covers several instances. This will guide you via a set of good coding practices for effectively using the Python return statement. Besides, these practices will help you put down more readable, manageable, strong, and efficient functions within Python.
Returning None Explicitly
Some coders rely on the “implicit return statement” thinking that Python will add any function without an explicit code. This can be confusing for developers who got knowledge of other coding languages. In those languages, a function without a return value is known as a procedure.
There are conditions where users can add an explicit return value as “None” to your functions. In other situations, however, users can rely on default Python behavior.
In case, the function executes actions but doesn’t have an explicit and useful return value, then users can exclude returning “None”. Doing that would be excess and confusing. Thus, users can also use an empty return without a return value. This is useful to make clear their purpose of returning from the function.
Moreover, suppose the function has several return statements. And if returning “None” is a valid option then users should consider the clear use of returning “None”. This is instead of relying on Python’s default action in Python return Statement.
These best practices can scale up the readability and maintainability of the user’s code by explicitly communicating their objective.
When it comes to returning “None” as a value, the user can use one of the three possible approaches that are the following:
- Omit or remove the return statement and base on the default Python behavior of returning “null” value.
- Use an empty return without a return value, which also returns None/Null in the outcome.
- Return None or null value very clearly.
Here’s how this works in practice:
Syntax
>>> def omit_return_stmt(): |
Whether to return “None” explicitly or not is the user’s personal decision. Although, users should observe that in some cases, an explicit return “None” value can avoid manageability problems. This is especially true in the case of developers who come from other coding languages backgrounds. Those languages don’t behave as Python does.
Recall the Return Value
While writing a custom function, users might forget by accident to return a value from a function. Here in the case, Python will return the “None” value for him in output. This can cause precise bugs that can be difficult for a new Python developer to understand and debug.
Thus, users can avoid this issue by writing the Python return statement right away after the header of the function. Then they can make another pass to write the function’s body section.
||{"title":"Master in Python", "subTitle":"Python Certification Training by ITGURU's", "btnTitle":"View Details","url":"https://onlineitguru.com/python-online-course","boxType":"reg"}||
Avoiding Complex Expressions
Earlier, it was a general practice to use the output of an expression as a return value within Python functions. Suppose, if the expression that the user is using becomes too complex. Then this practice can cause functions that are complex to understand, debug, and manage.
For example, if a user is doing a complex computation for a given input. Then it would be more readable to the regular increase in calculating the final result. He does so by using short-term variables with meaningful names.
In general, the user should evade using complex expressions within the return statement he prepares. Instead, the user can break the code into various stages and use short-term variables for each stage. Moreover, using the short-term variables can make the user code easier to debug, understand, and manage.
Using return Statements with “Conditionals”
Mostly Python functions are not confined to include a single return statement. In case the input function includes more than one return statement. Then the first one came across will ascertain the end of the function’s performance and also its return value.
The general way of coding functions with multiple return statements is the usage of conditional statements. This allows users to provide several return statements depending on the output of assessing some conditions.
Suppose, a user needs to write a function that captures a number and returns its exact value. In case, the number or value is greater than “zero”, then the user will return the same value. If in case, the number is less than “zero”, then the user will return its opposite or non-negative value.
Summing up
Thus, using a Python return Statement users can check the return value or output of a function. Along with this, they can end up executing it easily. We reach to conclude the topic in the Python return statement and its overview. I tried to explain things in a clear and simple manner. And I hope you got the overall idea of the Python return statement (s) and their usage. To get more insights on Python language, go through the Python Online Training. This may help you to achieve your career goals with expert skills.