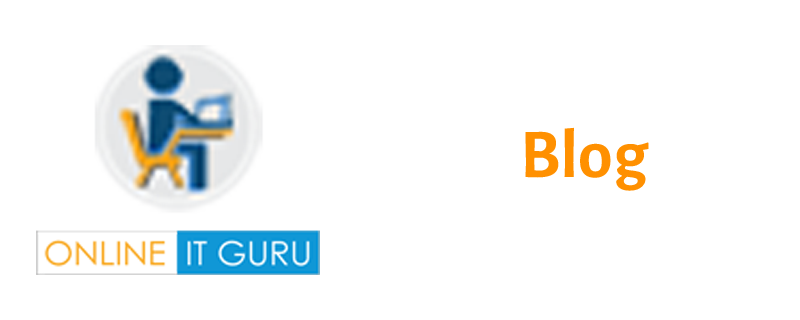
The Python requests module allows the user to exchange HTTP requests on the web. It’s a most useful library that helps to send HTTP requests including various methods and features. HTTP is a kind of request-response system that acts between the client and server where the client browser requests server to access it. Here, the web browser is a client and the system that hosts the website is a server.
Moreover, we will deep dive into the various Python requests modules and the types of requests made using Python.
Python requests module
To get started with Python requests we need to install them first. The following way is useful to install the requests.
$ pip install requests
After installing the requests module, we will check into the methods that are useful to request a response from the server. These are;
GET is useful to request any data from the server.
POST is useful to submit the data to be processed to the server.
Now, in the Python requests module, we need to use some HTTP libraries to make the HTTP requests. These are; httlib, urllib & requests. Here, we will use the requests library. Later, we will look into making a Get request. This is the most common method of requests. The syntax given below will help to get it.
# importing the requests library import requests # api-endpoint URL = "http://maps.googleapis.com/maps/api/geocode/json" # location given here location = "delhi technological university" PARAMS = {'address':location} r = requests.get # extracting data in json format data = r.json() # extracting latitude, longitude and formatted address # of the first matching location latitude = data['results'][0]['geometry']['location']['lat'] longitude = data['results'][0]['geometry']['location']['lng'] formatted_address = data['results'][0]['formatted_address'] # printing the output print("Latitude:%s\nLongitude:%s\nFormatted Address:%s" %(latitude, longitude,formatted_address)) |
The above example refers to sending and getting the request from the server at the request point. Here, the Python requests library parameters defined as a dictionary. Moreover, we create a response object that helps to store the request-response getting from the server. Besides, to retrieve the data from the response object, we need to convert the raw data into JSON type data.
Get practical insights into using Python from Python Online Course from the industry experts.
After the Get requests, now we make the POST request. The following command will help to send the Post request.
# importing the requests library import requests # defining the api-endpoint API_ENDPOINT = "http://pastebin.com/api/api_post.php" # your API key here API_KEY = "XXXXXXXXXXXXXXXXX" # your source code here source_code = ''' print("Hello, world!") a = 1 b = 2 print(a + b) ''' # data to be sent to api data = {'api_dev_key':API_KEY, 'api_option':'paste', 'api_paste_code':source_code, 'api_paste_format':'python'} r = requests.post # extracting response text pastebin_url = r.text print("The pastebin URL is:%s"%pastebin_url) |
Here, the above commands have taken for example. They explain the process of sending a Post request. Moreover, making a post request we will get the processed data that we look for from any URL. The Post request doesn’t contain any restriction on its data length. Thus, these are suitable for requesting files and images as well.
The Python requests Post method is useful for several other tasks also like filling and submitting any web forms, and postings to social media, etc.
HEAD Method
The requests library is one of the major aspects of Python language to make HTTP requests to a particular URL. We have discussed the GET and POST methods of Python requests earlier. Now we will discuss the “HEAD request” to a specific URL using requests.head() method. Before we look at the making HEAD method request, let’s check out what an “Http HEAD request” is:-
HEAD Http Method
The “HEAD HTTP” request method has HTTP support and widely used by the World Wide Web (www). The HEAD http method requests a response similar to that of a “GET request”. But this request is performed without the response body. This method is useful for recovering meta-data written in response headers, without having to transmit the whole content.
Making a HEAD request method using Python requests
The “Python requests module” offers an in-built method called head() to make “HEAD requests” to a particular URL.
The syntax for this request –
requests.head(url, params={key: value}, args)
DELETE Method
Using the “Delete request method,” check how to make a DELETE request to a particular URL with requests.delete(). Before we check the use of the “DELETE method”, let’s see what an “Http DELETE” request is –
DELETE Http Method
The “DELETE http” is a method of request in Python requests with the support of HTTP useful for the World Wide Web. This method mainly deletes or removes the particular resource of any data. Using a “PUT” request, users need to define a specific resource for this activity.
||{"title":"Master in Python", "subTitle":"Python Certification Training by ITGURU's", "btnTitle":"View Details","url":"https://onlineitguru.com/python-online-course","boxType":"demo","videoId":"Qtdzdhw6JOk"}||
Here, we can see the response status. In case, the response includes an entity defining the status, a successful response “SHOULD be 200 (OK)”. The response “202 (Accepted)” refers to, in case the action has not yet been validated. or response “204 (No Content)” in case the action has been performed but the response doesn’t consist of an entity.
Making Delete request using Python requests
Here, Python requests module provides an in-built method called delete() to make a DELETE request to a particular URL.
For this method, the Syntax – requests.delete(url, params={key: value}, args)
Response Types
The type of response object in the Python requests modules can be a byte, string, or JSON type file. Moreover, the users only allow reading a specific number of bytes by reading raw values in response.
Authentication of request
Authentication is necessary for any requests made by the users. Besides, this required several APIs that allow access to the user for specific details. Moreover, these requests support many types of authentications such as basic Auth and digest Auth.
The Session Object
Since we have seen the dealing with high level requests APIs such as GET() and POST(). these functions are the abstractions of the results upon making the requests. They usually hide the deployment details such as the working of several connections that manage requests, etc.
Moreover, those abstractions are called Session. To put our control over the requests being made or to improve the performance of the requests made, we need to use a Session instance directly in this module. The primary role of the session is performance optimization of a session. Moreover, it comes in the form of several connections. For example, when our application makes a connection or requests to a server using a Session, it keeps that request within a connection pool.
import requestsfrom getpass import getpasswith requests.Session() as session: session.auth = ('username', getpass()) response = session.get('https://api.github.com/user')print(response.headers)print(response.json())
Moreover, some browsers use cookies that are useful to store the user ID and maintain the login session of the user. These are very small pieces of data that are usually stored on the client’s browser. Besides, there are some other features also like timeout and redirection. The timeout feature allows the requests to terminate the session upon expiration. Besides, it usually happens when there is no proper response from the browser on any requests sent. Finally, the browser redirects the session where we have started. This is a default action of any browser at the time of session failure.
Python requests using Rest API
Python includes a simple syntax language that makes it easier to interact with REST APIs. Moreover, the Python requests library has different special functionality. Before we know how to perform Python requests using the REST API, we will try to know about the HTTP, APIs, and the REST features.
An API is a type of web service that gives permission to access particular data and methods. This is similar to accessing other apps and sometimes editing them through standard HTTP protocols, just like a website. Besides, this makes it easy to integrate APIs much faster into a different range of applications. REST refers to “Representational State Transfer” which is likely the most common architectural style of APIs for web services. It includes a set of guidelines and protocols that intend to simplify client/server communication. Furthermore, the REST APIs make data access much easier, simple, and logical.
Request
When a user interacts with data using a REST API, then it is called a “request”. Generally, a request includes the following components;
Endpoint
Data
Method
Headers
Endpoint
The endpoint is the URL that describes the data with which a user is going to interact. Besides, this is similar to tying up a particular page to a web page URL. Like that, an endpoint URL is also tied to a particular resource under an API.
Data
In case a user is using a method that includes data modification within a REST API, then he needs to include a data payload with the request. Moreover, this involves all the data that will be developed or changed.
Method
The method defines how users are connecting with the resource located at the given endpoint. REST APIs can offer various methods to allow full- {Create, Read, Update, and Delete} (CRUD) functionality. Moreover, the below are common methods that most REST APIs offer;
GET
POST
PUT
DELETE
Headers
The “headers” include any metadata that needs to be involved with the request. Like authentication tokens, the type of content has to be returned, and any other caching policies.
In the same way, the response is the return form of a reply when a user makes any request.
The rest of the method of “Python requests” is similar as we discussed above in this article.
Handling HTTP errors with Python requests
All API calls don’t always go as per the plan, some of them may fail. Moreover, there are different thoughts on the reasons why API requests might fail sometimes. This could be the defect of either the server or the client while making any Python requests. If the user is going to use a REST API, then he must need to know about handling the errors they output. It is important when things go wrong to make the code more robust/strong. Thus, we had seen a brief on handling HTTP errors with Python Requests.
HTTP Status Codes basics
In the context of Python Requests, we also need to get back to understand what HTTP status codes are? How they relate to errors that a user might encounter while performing requests.
These status codes fall under five different categories. Such as;
- Informational – This mentions that a request has been received and the client can still make the Python requests for the information payload. Users probably won’t go to worry about these status codes when they are working with Python Requests.
- Successful– This category means that a requested action has been received, understood, and accepted. Besides, users can use these codes to confirm the existence of information before attempting to act thereon.
- Redirection– This means the client must take further action to finish the request. Like acquiring the resource through a proxy or a special endpoint. Users may go to make any further requests or change their Python requests to affect these codes.
- Client Error– The category refers to issues with the client, like a scarcity of authorization, prohibited access, disallowed methods, or attempts to access imagined resources. Moreover, this generally refers to the configuration errors on the client application.
- Server Error– This means the issues with the server that gives the API. There is an outsized sort of server errors and that they often need the API provider to solve the issues.
Important points to remember in the Python requests module
Here, while using the GET method, all the form data is encoded into the URL. Later, this is attached to the URL that acts as a query string parameter. Moreover, using POST request, the form data usually appears in the message body of the HTTP request that the user makes.
Under the GET method in Python requests modules, the parameter data is limited to infuse into the request line or the URL. It is safe to use fewer parameters, as some servers couldn’t handle much data. Moreover, there is no such kind of problem in the POST method. Here, we should note that we are sending data into the message body of the HTTP requests and not the URL.
||{"title":"Master in Python", "subTitle":"Python Certification Training by ITGURU's", "btnTitle":"View Details","url":"https://onlineitguru.com/python-online-course","boxType":"reg"}||
In the GET method or request, only ASCII characters are allowed for sending data. But there is no such type of restriction in the POST request module.
Furthermore, the GET request is less secure in comparison to the POST request. Because here the data sent as the request is a part of the URL. Thus, the GET method is not much useful while sending requests for passwords or other sensitive information.
In programming, a library is a collection of some routines, functions, and operations that a program uses to perform various activities. Besides, these elements or objects are the modules. The libraries or requests are important because the user loads a module or request and takes advantage of everything. Moreover, it offers the results without linking to the program that relies on them. These are truly standalone modules. Using them we can build their own programs and also they remain isolated from the other programs.
Conclusion
Thus, the above details explain the Python requests module ad its various aspects. Using these requests any user can request any data or information from the server by placing an order. Moreover, there are many uses of Python requests. The users get to know how to make requests properly towards the web host or the server. Moreover, the HTTP requests play an important role in these request sessions. Using them, any user can get his required data.
Get more knowledge on Python requests and other modules through Python Online Training from the expert voice of IT Guru. This learning may help to enhance skills in this regard to plan for a better future.